(1)官方下載ShareSDK iOS 2.8.8,地址:http://sharesdk.cn/
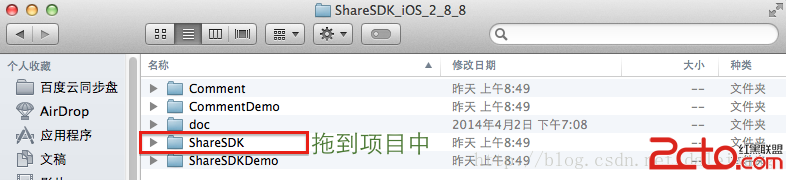
(2)根據實際情況,引入相關的庫,參考官方文檔。
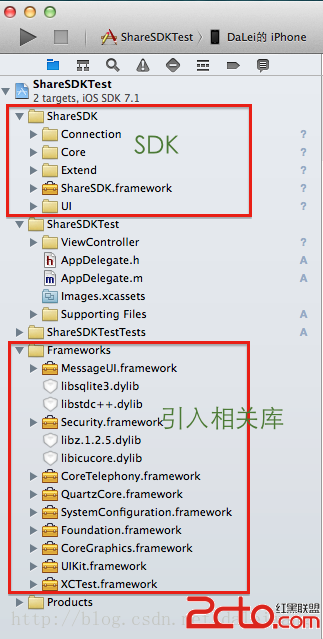
(3)在項目的AppDelegate中一般情況下有三個操作,第一是注冊ShareSDK,第二是注冊各個平台的賬號,第三是關於微信等應用的回調處理。
- //
- // AppDelegate.m
- // ShareSDKTest
- //
- // Created by wangdalei on 14-6-23.
- // Copyright (c) 2014年 王大雷. All rights reserved.
- //
-
- #import AppDelegate.h
- #import RootViewController.h
- #import
- #import WeiboApi.h
- #import
- #import
- #import WXApi.h
- #import
- #import
-
- @implementation AppDelegate
- @synthesize rootVC;
-
- - (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
- self.window = [[UIWindow alloc] initWithFrame:[[UIScreen mainScreen] bounds]];
- if (self.rootVC==nil) {
- self.rootVC = [[RootViewController alloc]initWithNibName:@RootViewController bundle:nil];
- }
- UINavigationController *rootNav = [[UINavigationController alloc]initWithRootViewController:self.rootVC];
- self.window.rootViewController = rootNav;
- self.window.backgroundColor = [UIColor whiteColor];
- [self.window makeKeyAndVisible];
-
-
- [ShareSDK registerApp:@1a2e7ab5fb6c];
-
- //添加新浪微博應用 注冊網址 http://open.weibo.com [email protected] 此處需要替換成自己應用的
- [ShareSDK connectSinaWeiboWithAppKey:@3201194191
- appSecret:@0334252914651e8f76bad63337b3b78f
- redirectUri:@http://appgo.cn];
-
- //添加騰訊微博應用 注冊網址 http://dev.t.qq.com [email protected] 此處需要替換成自己應用的
- [ShareSDK connectTencentWeiboWithAppKey:@801307650
- appSecret:@ae36f4ee3946e1cbb98d6965b0b2ff5c
- redirectUri:@http://www.sharesdk.cn
- wbApiCls:[WeiboApi class]];
-
- //添加QQ空間應用 注冊網址 http://connect.qq.com/intro/login/ [email protected] 此處需要替換成自己應用的
- [ShareSDK connectQZoneWithAppKey:@100371282
- appSecret:@aed9b0303e3ed1e27bae87c33761161d
- qqApiInterfaceCls:[QQApiInterface class]
- tencentOAuthCls:[TencentOAuth class]];
-
- //此參數為申請的微信AppID [email protected] 此處需要替換成自己應用的
- [ShareSDK connectWeChatWithAppId:@wx4868b35061f87885 wechatCls:[WXApi class]];
-
- //添加QQ應用 該參數填入申請的QQ AppId [email protected] 此處需要替換成自己應用的
- [ShareSDK connectQQWithQZoneAppKey:@100371282
- qqApiInterfaceCls:[QQApiInterface class]
- tencentOAuthCls:[TencentOAuth class]];
-
- return YES;
- }
-
-
- - (void)applicationWillResignActive:(UIApplication *)application {
- // Sent when the application is about to move from active to inactive state. This can occur for certain types of temporary interruptions (such as an incoming phone call or SMS message) or when the user quits the application and it begins the transition to the background state.
- // Use this method to pause ongoing tasks, disable timers, and throttle down OpenGL ES frame rates. Games should use this method to pause the game.
- }
-
- - (void)applicationDidEnterBackground:(UIApplication *)application {
- // Use this method to release shared resources, save user data, invalidate timers, and store enough application state information to restore your application to its current state in case it is terminated later.
- // If your application supports background execution, this method is called instead of applicationWillTerminate: when the user quits.
- }
-
- - (void)applicationWillEnterForeground:(UIApplication *)application {
- // Called as part of the transition from the background to the inactive state; here you can undo many of the changes made on entering the background.
- }
-
- - (void)applicationDidBecomeActive:(UIApplication *)application {
- // Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
- }
-
- - (void)applicationWillTerminate:(UIApplication *)application {
- // Called when the application is about to terminate. Save data if appropriate. See also applicationDidEnterBackground:.
- }
-
-
- #pragma mark - WX回調
-
- - (BOOL)application:(UIApplication *)application handleOpenURL:(NSURL *)url {
- return [ShareSDK handleOpenURL:url wxDelegate:self];
- }
-
- - (BOOL)application:(UIApplication *)application openURL:(NSURL *)url sourceApplication:(NSString *)sourceApplication annotation:(id)annotation {
- return [ShareSDK handleOpenURL:url sourceApplication:sourceApplication annotation:annotation wxDelegate:self];
- }
-
- #pragma mark - WXApiDelegate
-
- /*! @brief 收到一個來自微信的請求,第三方應用程序處理完後調用sendResp向微信發送結果
- *
- * 收到一個來自微信的請求,異步處理完成後必須調用sendResp發送處理結果給微信。
- * 可能收到的請求有GetMessageFromWXReq、ShowMessageFromWXReq等。
- * @param req 具體請求內容,是自動釋放的
- */
- -(void) onReq:(BaseReq*)req{
-
- }
-
- /*! @brief 發送一個sendReq後,收到微信的回應
- *
- * 收到一個來自微信的處理結果。調用一次sendReq後會收到onResp。
- * 可能收到的處理結果有SendMessageToWXResp、SendAuthResp等。
- * @param resp具體的回應內容,是自動釋放的
- */
- -(void) onResp:(BaseResp*)resp{
-
- }
-
- @end
(4)信息分享。
- -(IBAction)share:(id)sender{
- NSString *imagePath = [[NSBundle mainBundle] pathForResource:@card ofType:@png];
- //構造分享內容
- id publishContent = [ShareSDK content:@分享內容測試
- defaultContent:@默認分享內容測試,沒內容時顯示
- image:[ShareSDK imageWithPath:imagePath]
- title:@pmmq
- url:@http://www.sharesdk.cn
- description:@這是一條測試信息
- mediaType:SSPublishContentMediaTypeNews];
- [ShareSDK showShareActionSheet:nil
- shareList:nil
- content:publishContent
- statusBarTips:YES
- authOptions:nil
- shareOptions: nil
- result:^(ShareType type, SSResponseState state, id statusInfo, id error, BOOL end) {
- if (state == SSResponseStateSuccess)
- {
- NSLog(@分享成功);
- }
- else if (state == SSResponseStateFail)
- {
- NSLog(@分享失敗);
- }
- }];
- }
(5)登錄、登出、獲取授權信息、關注制定微博
- //
- // LoginViewController.m
- // ShareSDKTest
- //
- // Created by wangdalei on 14-6-23.
- // Copyright (c) 2014年 王大雷. All rights reserved.
- //
-
- #import LoginViewController.h
- #import
-
- @interface LoginViewController ()
-
- -(IBAction)loginWithSina:(id)sender;
-
- -(IBAction)loginWithQQ:(id)sender;
-
- -(IBAction)loginoutWithSina:(id)sender;
-
- -(IBAction)loginoutWithQQ:(id)sender;
-
- -(IBAction)guanzhuUs:(id)sender;
-
- -(void)reloadStateWithType:(ShareType)type;
-
- @end
-
- @implementation LoginViewController
-
- - (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil {
- self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
- if (self) {
- }
- return self;
- }
-
- - (void)viewDidLoad {
- [super viewDidLoad];
- }
-
- - (void)didReceiveMemoryWarning {
- [super didReceiveMemoryWarning];
- }
-
- - (IBAction)loginWithSina:(id)sender {
- [ShareSDK getUserInfoWithType:ShareTypeSinaWeibo authOptions:nil result:^(BOOL result, id userInfo, id error) {
- NSLog(@%d,result);
- if (result) {
- //成功登錄後,判斷該用戶的ID是否在自己的數據庫中。
- //如果有直接登錄,沒有就將該用戶的ID和相關資料在數據庫中創建新用戶。
- [self reloadStateWithType:ShareTypeSinaWeibo];
- }
- }];
- }
-
-
- -(IBAction)loginWithQQ:(id)sender{
- [ShareSDK getUserInfoWithType:ShareTypeQQSpace authOptions:nil result:^(BOOL result, id userInfo, id error) {
- NSLog(@%d,result);
- if (result) {
- //成功登錄後,判斷該用戶的ID是否在自己的數據庫中。
- //如果有直接登錄,沒有就將該用戶的ID和相關資料在數據庫中創建新用戶。
- [self reloadStateWithType:ShareTypeQQSpace];
- }
- }];
- }
-
- -(IBAction)loginoutWithSina:(id)sender{
- [ShareSDK cancelAuthWithType:ShareTypeSinaWeibo];
- [self reloadStateWithType:ShareTypeSinaWeibo];
- }
-
- -(IBAction)loginoutWithQQ:(id)sender{
- [ShareSDK cancelAuthWithType:ShareTypeQQSpace];
- [self reloadStateWithType:ShareTypeQQSpace];
- }
-
- -(void)reloadStateWithType:(ShareType)type{
- //現實授權信息,包括授權ID、授權有效期等。
- //此處可以在用戶進入應用的時候直接調用,如授權信息不為空且不過期可幫用戶自動實現登錄。
- id credential = [ShareSDK getCredentialWithType:type];
- UIAlertView *alertView = [[UIAlertView alloc] initWithTitle:NSLocalizedString(@TEXT_TIPS, @提示)
- message:[NSString stringWithFormat:
- @uid = %@ token = %@ secret = %@ expired = %@ extInfo = %@,
- [credential uid],
- [credential token],
- [credential secret],
- [credential expired],
- [credential extInfo]]
- delegate:nil
- cancelButtonTitle:NSLocalizedString(@TEXT_KNOW, @知道了)
- otherButtonTitles:nil];
- [alertView show];
- }
-
- //關注用戶
- -(IBAction)guanzhuUs:(id)sender{
- [ShareSDK followUserWithType:ShareTypeSinaWeibo //平台類型
- field:@ShareSDK //關注用戶的名稱或ID
- fieldType:SSUserFieldTypeName //字段類型,用於指定第二個參數是名稱還是ID
- authOptions:nil //授權選項
- viewDelegate:nil //授權視圖委托
- result:^(SSResponseState state, id userInfo, id error) {
- if (state == SSResponseStateSuccess) {
- NSLog(@關注成功);
- } else if (state == SSResponseStateFail) {
- NSLog(@%@, [NSString stringWithFormat:@關注失敗:%@, error.errorDescription]);
- }
- }];
- }
-
-
- @end
(5)你可能會看到一些應用需要第三方登錄的,一種是彈出webView加載的新浪微博或者qq的網頁授權,還有一種是跳轉到本地的已經安裝的新浪微博應用或者qq應用進行授權。第二種授權方式較SSO授權,體驗會比較好一些,因為不需要用戶輸入新浪微博或QQ的用戶名與密碼。
第二種授權方式需要在plist中配置Scheme。SSO默認是打開的不需要配置。在AppDelegate中實現回調。
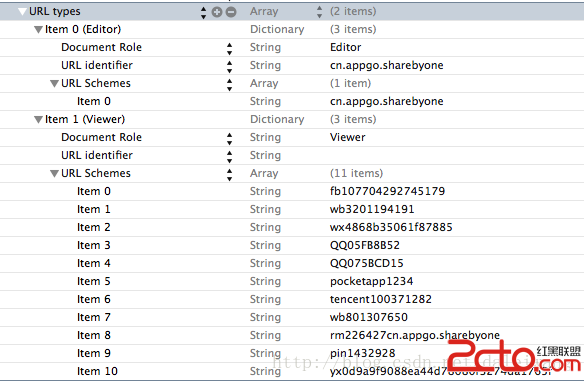
(6)測試DEMO截圖:
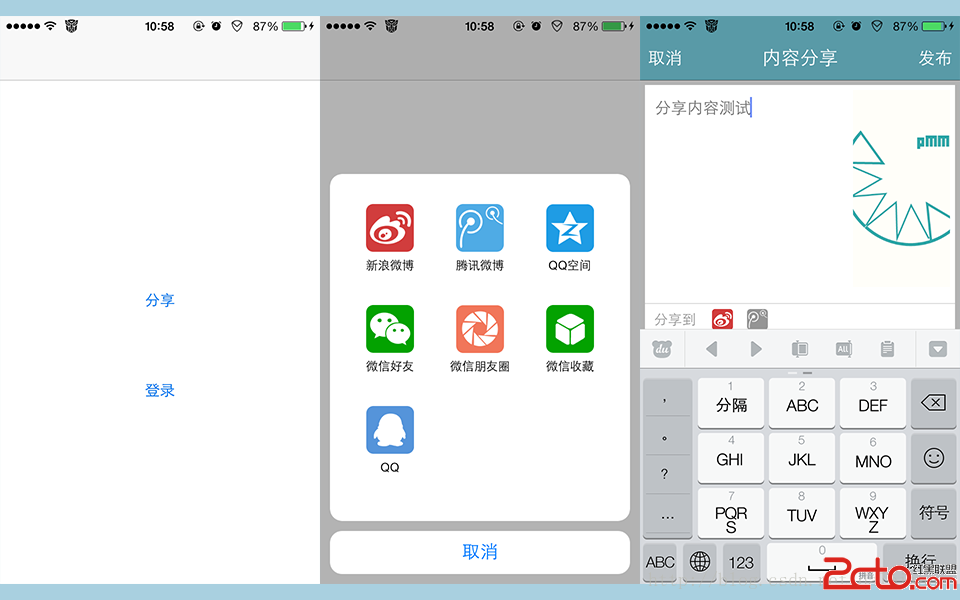
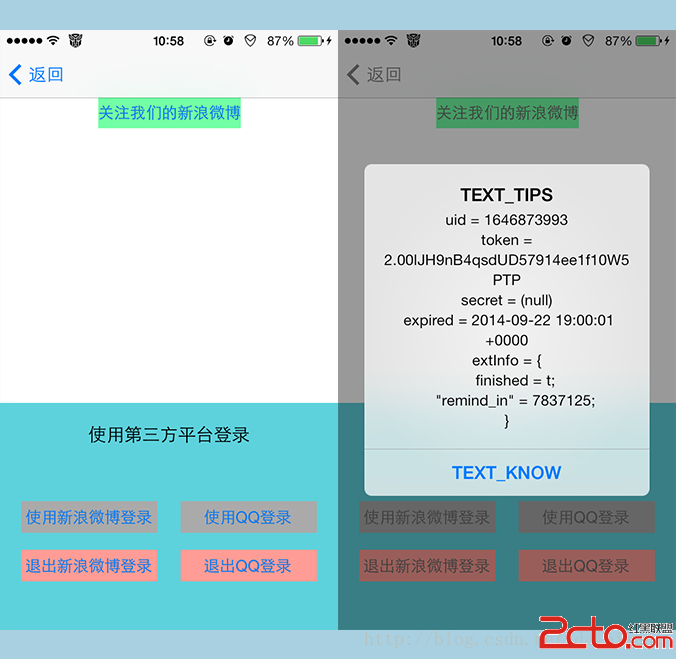